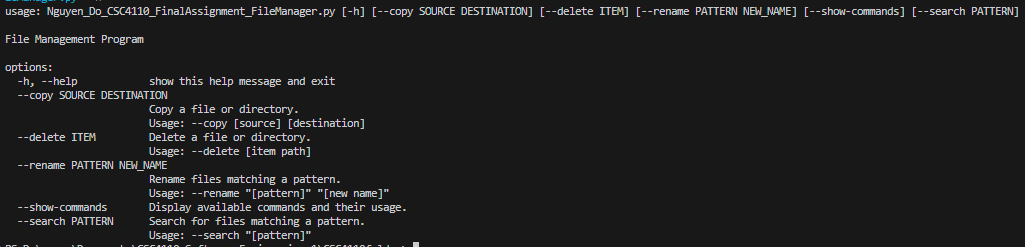
import argparse
import os
import shutil
import datetime
import re
# Log file operations to system_log.txt.
def log_operation(operation):
with open("system_log.txt", "a") as log_file:
log_file.write(f"{datetime.datetime.now()}: {operation}\n")
# Copy files or directories and log the operation.
def copy_item(source, destination):
if os.path.isdir(source):
shutil.copytree(source, destination)
log_operation(f"Directory '{source}' was copied to '{destination}'")
else:
shutil.copy2(source, destination)
log_operation(f"File '{source}' was copied to '{destination}'")
# Delete files or directories after backing them up.
def delete_item(item):
backup_dir = "backups"
if not os.path.exists(backup_dir):
os.makedirs(backup_dir)
basename = os.path.basename(item)
backup_path = os.path.join(backup_dir, f"deleted_{basename}")
copy_item(item, backup_path)
if os.path.isdir(item):
shutil.rmtree(item)
else:
os.remove(item)
log_operation(f"'{item}' was deleted")
# Rename files matching a specific pattern.
def rename_files(search_pattern, new_name):
for root, dirs, files in os.walk('.'):
for file in files:
if re.search(search_pattern, file):
new_file_name = new_name + os.path.splitext(file)[1]
new_file_path = os.path.join(root, new_file_name)
# Conflict handling: Avoid overwriting existing files
counter = 1
while os.path.exists(new_file_path):
new_file_name = f"{new_name}_{counter}{os.path.splitext(file)[1]}"
new_file_path = os.path.join(root, new_file_name)
counter += 1
os.rename(os.path.join(root, file), new_file_path)
log_operation(f"File '{file}' was renamed to '{new_file_name}'")
# Search for files matching a specific pattern.
def search_files(search_pattern):
"""Search for files containing a specific substring."""
found_files = []
for root, dirs, files in os.walk('.'):
for file in files:
if search_pattern in file: # Simple substring check
found_files.append(os.path.join(root, file))
return found_files
def main():
# Parse command-line arguments and perform file operations.
parser = argparse.ArgumentParser(description="File Management Program",
formatter_class=argparse.RawTextHelpFormatter)
parser.add_argument("--copy", nargs=2, metavar=('SOURCE', 'DESTINATION'),
help="Copy a file or directory.\nUsage: --copy [source] [destination]")
parser.add_argument("--delete", metavar='ITEM',
help="Delete a file or directory.\nUsage: --delete [item path]")
parser.add_argument("--rename", nargs=2, metavar=('PATTERN', 'NEW_NAME'),
help="Rename files matching a pattern.\nUsage: --rename \"[pattern]\" \"[new name]\"")
parser.add_argument("--show-commands", action="store_true",
help="Display available commands and their usage.")
parser.add_argument("--search", metavar='PATTERN',
help="Search for files matching a pattern.\nUsage: --search \"[pattern]\"")
args = parser.parse_args()
if args.show_commands:
parser.print_help()
return
if args.copy:
source, destination = args.copy
copy_item(source, destination)
if args.delete:
delete_item(args.delete)
if args.rename:
pattern, new_name = args.rename
rename_files(pattern, new_name)
if args.search:
pattern = args.search
matching_files = search_files(pattern)
if matching_files:
print("Found files:")
for file in matching_files:
print(file)
else:
print("No files found matching the pattern.")
if __name__ == "__main__":
main()